Coroutines based Kotlin interview questions and answers
What are kotlin coroutines?
Kotlin's coroutines are lightweight threads. It provides a good way to write asynchronous code that is perfectly readable and maintainable. Kotlin provides only minimal low-level APIs in its standard library to enable various other libraries to utilize coroutines.
What are coroutines in Kotlin? What are coroutines in Kotlin?
Unlike many other languages with similar capabilities, async and await are not keywords in Kotlin and are not even part of its standard library.
kotlinx.coroutines
is a rich library for coroutines developed by JetBrains. It contains a number of high-level coroutine-enabled primitives, including launch
, async
and others. Kotlin Coroutines give you an API to write your asynchronous code sequentially.
The documentation says Kotlin Coroutines are like lightweight threads. They are lightweight because creating coroutines doesn’t allocate new threads. Instead, they use predefined thread pools, and smart scheduling. Scheduling is the process of determining which piece of work you will execute next.
Additionally, coroutines can be suspended and resumed mid-execution. This means you can have a long-running task, which you can execute little-by-little. You can pause it any number of times, and resume it when you’re ready again.
What is the difference between suspending vs. blocking? What is the difference between suspending vs. blocking?
A blocking call to a function means that a call to any other function, from the same thread, will halt the parent’s execution. Following up, this means that if you make a blocking call on the main thread’s execution, you effectively freeze the UI. Until that blocking calls finishes, the user will see a static screen, which is not a good thing.
Suspending doesn’t necessarily block your parent function’s execution. If you call a suspending function in some thread, you can easily push that function to a different thread. In case it is a heavy operation, it won’t block the main thread. If the suspending function has to suspend, it will simply pause its execution. This way you free up its thread for other work. Once it’s done suspending, it will get the next free thread from the pool, to finish its work.
What is Coroutine Scope and how is that different from Coroutine Context? What is Coroutine Scope and how is that different from Coroutine Context?
Coroutines always execute in some context represented by a value of the CoroutineContext type, defined in the Kotlin standard library. The coroutine context is a set of various elements. The main elements are the Job of the coroutine.
CoroutineScope has no data on its own, it just holds a CoroutineContext. Its key role is as the implicit receiver of the block you pass to
launch
,async
etc.runBlocking { val scope0 = this // scope0 is the top-level coroutine scope. scope0.launch { val scope1 = this // scope1 inherits its context from scope0. It replaces the Job field // with its own job, which is a child of the job in scope0. // It retains the Dispatcher field so the launched coroutine uses // the dispatcher created by runBlocking. scope1.launch { val scope2 = this // scope2 inherits from scope1 } } }
You might say that CoroutineScope formalizes the way the CoroutineContext is inherited. You can see how the CoroutineScope mediates the inheritance of coroutine contexts. If you cancel the job in
scope1
, this will propagate toscope2
and will cancel the launched job as well.
How do you launch a coroutine in Kotlin?
Call the launch function on the CoroutineScope object to launch a coroutine, passing in the function you wish to execute. This will create a new coroutine and launch it immediately.
How do you cancel a coroutine in Kotlin?
Call the cancel function on the CoroutineScope object to cancel a coroutine in Kotlin. This will cancel the coroutine and free up any resources.
How do you pass data between coroutines in Kotlin?
One of the best ways to pass data between coroutines in Kotlin is with channel objects, which allow for safe and synchronized communication between separate threads or processes. To create a channel object, simply use the Channel constructor and define any channels you want to send data through.
What are Coroutines in Kotlin?
A framework to manage concurrency in a more performant and simple way with its lightweight thread which is written on top of the actual threading framework to get the most out of it by taking the advantage of cooperative nature of functions.
This is an important interview question.
Learn more about Kotlin Coroutines with examples from MindOrks blog.
What is suspend function in Kotlin Coroutines?
Suspend function is the building block of the Coroutines in Kotlin. Suspend function is a function that could be started, paused, and resume. To use a suspend function, we need to use the suspend keyword in our normal function definition.
Learn more about the suspend function from MindOrks blog.
What is the difference between Launch and Async in Kotlin Coroutines?
The difference is that the launch{}
does not return anything and the async{}
returns an instance of Deferred<T>
, which has an await()
function that returns the result of the coroutine like we have future in Java in which we do future.get()
to the get the result.
In other words:
- launch: fire and forget
- async: perform a task and return a result
Learn more about Launch vs Async from MindOrks video
What are scopes in Kotlin Coroutines?
How Exception Handling is done in Kotlin Coroutines?
Explain suspend function in the context of Kotlin.
A function that may be started, halted, then resumed is known as a suspend function. One of the most important things to remember about the suspend functions is that they can only be invoked from another suspend function or from a coroutine. Suspending functions are merely standard Kotlin functions with the suspend modifier added, indicating that they can suspend coroutine execution without blocking the current thread. This means that the code you're looking at may pause execution when it calls a suspending function and restart execution at a later time. However, it makes no mention of what will happen to the present thread in the meantime.
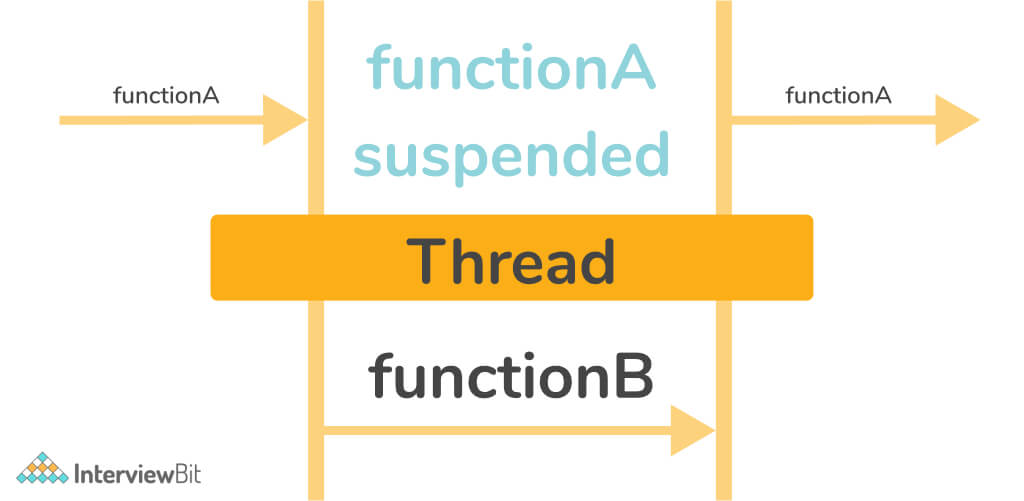
Suspending functions can call any other ordinary functions, but another suspending function is required to suspend the execution. Because a suspending function cannot be called from a regular function, numerous coroutine builders are supplied, allowing you to call a suspending function from a non-suspending scope like launch, async, or runBlocking.
delay() function is an example of suspend function.
What is suspending function in Kotlin?
A suspending function is just a regular Kotlin function with an additional suspend modifier which indicates that the function can suspend the execution of a coroutine without blocking the current thread. This means that the code you are looking at might stop executing at the moment it calls a suspending function, and will resume at some later time. However, it doesn’t say anything about what the current thread will do in the meantime.
Suspending functions can invoke any other regular functions, but to actually suspend the execution, it has to be another suspending function.A suspending function cannot be invoked from a regular function, therefore several so-called coroutine builders are provided, which allow calling a suspending function from a regular non-suspending scope like launch
, async
, runBlocking
What is the difference between suspending vs. blocking?
- A blocking call to a function means that a call to any other function, from the same thread, will halt the parent’s execution. Following up, this means that if you make a blocking call on the main thread’s execution, you effectively freeze the UI. Until that blocking calls finishes, the user will see a static screen, which is not a good thing.
- Suspending doesn’t necessarily block your parent function’s execution. If you call a suspending function in some thread, you can easily push that function to a different thread. In case it is a heavy operation, it won’t block the main thread. If the suspending function has to suspend, it will simply pause its execution. This way you free up its thread for other work. Once it’s done suspending, it will get the next free thread from the pool, to finish its work.
What are scope functions in Kotlin?
The Kotlin standard library contains several functions whose sole purpose is to execute a block of code within the context of an object. When you call such a function on an object with a lambda expression provided, it forms a temporary scope. In this scope, you can access the object without its name. Such functions are called scope functions.
There are five of them:
let
,run
,with
,apply
,also
.
Differentiate between launch / join and async / await in Kotlin.
launch / join:-
The launch command is used to start and stop a coroutine. It's as though a new thread has been started. If the code inside the launch throws an exception, it's considered as an uncaught exception in a thread, which is typically written to stderr in backend JVM programs and crashes Android applications. Join is used to wait for the launched coroutine to complete before propagating its exception. A crashed child coroutine, on the other hand, cancels its parent with the matching exception.
async / await:-
The async keyword is used to initiate a coroutine that computes a result. You must use await on the result, which is represented by an instance of Deferred. Uncaught exceptions in async code are held in the resultant Deferred and are not transmitted anywhere else. They are not executed until processed.
Comments
Post a Comment