OOPs based Kotlin interview questions and answers
Class and Object:
Is new a keyword in Kotlin? How would you instantiate a class object in Kotlin?
NO. Unlike Java, in Kotlin, new isn’t a keyword.
We can instantiate a class in the following way:
class A
var a = A()
val new = A()
Tell me the default behavior of Kotlin classes?
In Kotlin all classes are final by default. That’s because Kotlin allows multiple inheritances for classes, and an open class is more expensive than a final class.
What are the class members in kotlin?
Ans: A class in kotlin have the following members:
- Initializer blocks
- Properties
- Open declarations
- Nested classes
- Inner classes
How do you define an object in Kotlin?
To define an object in Kotlin, simply declare a class and instantiate it with the new keyword. This will create a new class instance, which can perform various actions.
val newObject= object {
val one = "Hello"
val two = "World"
override fun toString() = "$one $two"
}
The above would print “Hello World.”
Init
init{
print("Class instance is initialized.") //gets executed when the object is created.
}
However, the priority for the init block is less than the primary constructor. So, if there is a primary constructor in a class, it gets executed first and then the init block. A class can have multiple init blocks in it.
What is init block in Kotlin
init
is the initialiser block in Kotlin. It’s executed once the primary constructor is instantiated. If you invoke a secondary constructor, then it works after the primary one as it is composed in the chain
OK. Is there something called init block in Kotlin ?
Yes.
What does init block do and Where does it appear in a class ? – Kotlin Interview Question
Instructions in the init block are executed right after Primary Constructor’s execution. init block goes in a class along with secondary constructors as a method.
Reference – Kotlin Init
What are init blocks in Kotlin?
init
blocks are initializer blocks that are executed just after the execution of the primary constructor. A class file can have one or more init blocks that will be executed in series. If you want to perform some operation in the primary constructor, then it is not possible in Kotlin, for that, you need to use the init
block.
What is the difference between a constructor and an initializer in Kotlin?
A constructor is a special method invoked when an object is created. An initializer is a special method you can use to initialize an object before its first use. Both constructors and initializers are typically declared with the unit keyword
Nested and Inner Class
Constructor :
What’s the type of arguments inside a constructor?
By default, the constructor arguments are val
unless explicitly set to var
.
val
unless explicitly set to var
.What are the types of constructors in Kotlin? How are they different? How do you define them in your class?
Constructors in Kotlin are of two types:
Primary – These are defined in the class headers. They cannot hold any logic. There’s only one primary constructor per class.
Secondary – They’re defined in the class body. They must delegate to the primary constructor if it exists. They can hold logic. There can be more than one secondary constructors.
class User(name: String, isAdmin: Boolean){
constructor(name: String, isAdmin: Boolean, age: Int) :this(name, isAdmin)
{
this.age = age
}
}
What is the type of arguments inside a constructor?
By default, the constructor arguments are val
unless explicitly set to var
.
Explain what is wrong with that code? Explain what is wrong with that code?
class Student (var name: String) {
init() {
println("Student has got a name as $name")
}
constructor(sectionName: String, var id: Int) this(sectionName) {
}
}
The property of the class can’t be declared inside the secondary constructor.. This will give an error because here we are declaring a property id
of the class in the secondary constructor, which is not allowed.
If you want to use some property inside the secondary constructor, then declare the property inside the class and use it in the secondary constructor:
class Student (var name: String) {
var id: Int = -1
init() {
println("Student has got a name as $name")
}
constructor(secname: String, id: Int) this(secname) {
this.id = id
}
}
How to create empty constructor for data class in Kotlin? How to create empty constructor for data class in Kotlin?
If you give default values to all the fields - empty constructor is generated automatically by Kotlin.
data class User(var id: Long = -1,
var uniqueIdentifier: String? = null)
and you can simply call:
val user = User()
Another option is to declare a secondary constructor that has no parameters:
data class User(var id: Long,
var uniqueIdentifier: String?){
constructor() : this(-1, null)
}
What are the types of constructors in Kotlin?
- Primary constructor: These constructors are defined in the class header and you can't perform some operation in it, unlike Java's constructor.
- Secondary constructor: These constructors are declared inside the class body by using the constructor keyword. You must call the primary constructor from the secondary constructor explicitly. Also, the property of the class can’t be declared inside the secondary constructor. There can be more than one secondary constructors in Kotlin.
Learn more about Primary and Secondary constructors from MindOrks blog.
Is there any relationship between primary and secondary constructors?
Yes, when using a secondary constructor, you need to call the primary constructor explicitly.
What is the default type of argument used in a constructor?
By default, the type of arguments of a constructor in val. But you can change it to var explicitly.
How are Primary Constructors different from Secondary Constructors ?
Primary Constructors are declared intrinsically with class definition. Secondary Constructors are declared exclusively inside the class body.
In the following example, in the first line, the constructor keyword along with the variables declared right after it is the Primary Constructor. Inside the class body, we have another constructor, and this is Secondary Constructor.
class Person constructor(var name: String, var age: Int){ var profession: String = "Not Mentioned" constructor (name: String, age: Int, profession: String): this(name,age){ this.profession = profession } } |
Q14 - Is there any dependency of Secondary Constructors on Primary Constructors ?
Yes. Secondary Constructor has to make an exclusive call to Primary Constructor or other Secondary Constructor, which of course calls the Primary Constructor. Following is an example, and here the Secondary Constructor makes call to Primary Constructor using this(name, age)
.
class Person constructor(var name: String, var age: Int){ var profession: String = "Not Mentioned" constructor (name: String, age: Int, profession: String): this(name,age){ this.profession = profession } fun printPersonDetails(){ println("$name whose profession is $profession, is $age years old.") } } |
If you give default values to all the primary constructor values, you shall automatically create an empty constructor for data class.
For example, if you have a data class like this:
data class Activity(
var updated_on: String,
var tags: List
var description: String,
var user_id: List
)
And you want to initialize the data class with an empty constructor, all you have to do is assign the default values to all the primary constructors, like this:
data class Activity(
var updated_on: String = "",
var tags: List
var description: String = "",
var user_id: List
)
What are the different types of constructors available in Kotlin? Explain them with proper examples.
There are two types of Kotlin constructors:
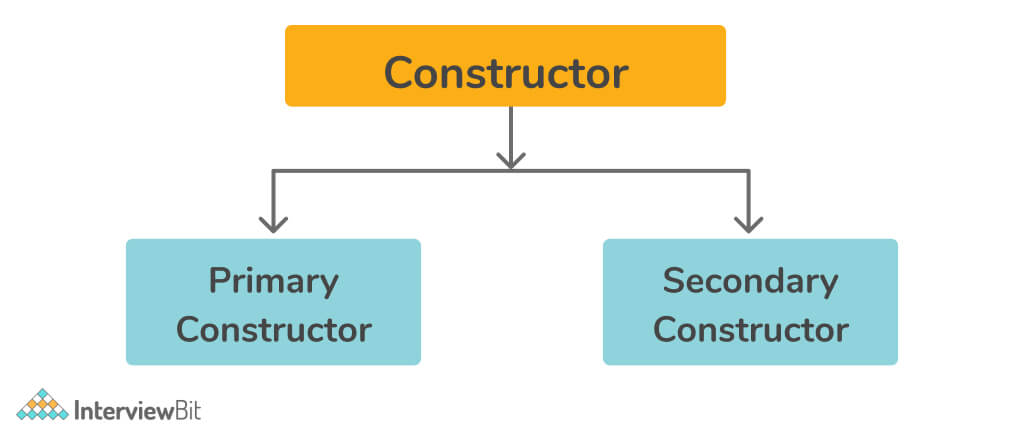
- Primary Constructor - This type of constructor is initialised in the class header and is provided after the class name. It is declared using the “constructor” keyword. Parameters are optional in this type of constructor. For example,
class Sample constructor(val a: Int, val b: Int) {
// code
}
If no annotations or access modifiers are provided, the constructor keyword can be omitted. The initialization code can be placed in a separate initializer block prefixed with the init keyword because the primary constructor cannot contain any code.
For example,
// KOTLIN
fun main(args: Array<String>) {
val s1 = Sample(1, 2)
}
class Sample(a : Int , b: Int) {
val p: Int
var q: Int
// initializer block
init {
p = a
q = b
println("The first parameter value is : $p")
println("The second parameter value is : $q")
}
}
Output:-
The first parameter value is: 1
The second parameter value is: 2
Explanation - The values 1 and 2 are supplied to the constructor arguments a and b when the object s1 is created for the class Sample. In the class p and q, two attributes are specified. The initializer block is called when an object is created, and it not only sets up the attributes but also prints them to the standard output.
- Secondary Constructor - Secondary constructors allow for the initialization of variables as well as the addition of logic to the class. They have the constructor keyword prefixed to them. For example,
// KOTLIN
fun main(args: Array<String>) {
val s1 = Sample(1, 2)
}
class Sample {
constructor(a: Int, b: Int) {
println("The first parameter value is : $p")
println("The second parameter value is : $q")
}
}
Output:-
The first parameter value is: 1
The second parameter value is: 2
The compiler determines which secondary constructor will be called based on the inputs provided. We don't specify which constructor to use in the above program, so the compiler chooses for us.
In Kotlin, a class can contain one or more secondary constructors and at most one primary constructor. The primary constructor initializes the class, while the secondary constructor initialises the class and adds some additional logic.
Visibility Modifier
List down the visibility modifiers available in Kotlin. What’s the default visibility modifier?
public
protected
private
internal
public is the default visibility modifier
What are visibility modifiers in Kotlin?
A visibility modifier or access specifier or access modifier is a concept that is used to define the scope of something in a programming language. In Kotlin, we have four visibility modifiers:
- private: visible inside that particular class or file containing the declaration.
- protected: visible inside that particular class or file and also in the subclass of that particular class where it is declared.
- internal: visible everywhere in that particular module.
- public: visible to everyone.
Note: By default, the visibility modifier in Kotlin is public.
Learn more about visibility modifiers in Kotlin from MindOrks blog.
Kotlin Inheritance
Does the following inheritance structure compile?
class A{
}
class B : A(){
}
NO. By default classes are final in Kotlin. To make them non-final, you need to add the open
modifier.
open class A{
}
class B : A(){
}
What is the difference between "open" and "public" in Kotlin? What is the difference between "open" and "public" in Kotlin?
Answer- The open keyword means “open for extension“. The open annotation on a class is the opposite of Java's
final
: it allows others to inherit from this class. - If you do not specify any visibility modifier, public is used by default, which means that your declarations will be visible everywhere. public is the default if nothing else is specified explicitly.
- The open keyword means “open for extension“. The open annotation on a class is the opposite of Java's
final
: it allows others to inherit from this class. - If you do not specify any visibility modifier, public is used by default, which means that your declarations will be visible everywhere. public is the default if nothing else is specified explicitly.
Is inheritance compile in Kotlin?
Formal inheritance structure does not compile in the kotlin. By using an open modifier we can finalize classes.
open class B
{
}
class c = B()
{
}
Formal inheritance structure does not compile in the kotlin. By using an open modifier we can finalize classes.
open class B
{
}
class c = B()
{
}
Abstract Class
What is the use of abstraction in Kotlin?
Abstraction is the most important concept of Objected Oriented Programming. In Kotlin, abstraction class is used when you know what functionalities a class should have. But you are not aware of how the functionality is implemented or if the functionality can be implemented using different methods.
What is the open keyword in Kotlin used for?
By default, the classes and functions are final in Kotlin. So, you can't inherit the class or override the functions. To do so, you need to use the open keyword before the class and function. For example:
Kotlin Interface
Data Class
What are data classes in Kotlin? What makes them so useful? How are they defined?
In Java, to create a class that stores data, you need to set the variables, the getters and the setters, override the toString()
, hash()
and copy()
functions.
In Kotlin you just need to add the data
keyword on the class and all of the above would automatically be created under the hood.
data class Book(var name: String, var
authorName: String)
fun main(args: Array<String>) {
val book = Book("Kotlin Tutorials","Anupam")
}
Thus, data classes saves us with lot of code.
It creates component functions such as component1()
.. componentN()
for each of the variables.
Explain the use of data class in Kotlin?
Data class holds the basic data types. It does not contain any functionality.
What are Data classes ? Aren’t they available in Java ?
Sometimes we use a class just to hold the data and nothing else. These classes are called Data classes. Of course these kind of classes could be built using Java, but with explicit implementation of getter and setter for each of the properties of class. Also you may need to implement functions like equals, toString and copy separately. What Kotlin does is implementing all these automatically along with special functions called component functions. How cool is that, removing the redundant code bloat.
Reference – Kotlin Data Class
What is the difference between the Java class and the kotlin data class?
The Kotlin language introduced the concept of Data Classes, which represent simple classes used as data containers. As the name indicates it is used to only store data and contains no other business logic.
Example
- public class Employee {
- private String empName;
- private int empId;
- public String getEmpName() {
- return empName;
- }
- public void setEmpName(String empName) {
- this.empName = empName;
- }
- public int getEmpId() {
- return empId;
- }
- public void setEmpId(int empId) {
- this.empId = empId;
- }
- @Override
- public int hashCode() {
- final int prime = 31;
- int result = 1;
- result = prime * result + empId;
- result = prime * result + ((empName == null) ? 0 : empName.hashCode());
- return result;
- }
- @Override
- public boolean equals(Object obj) {
- if (this == obj)
- return true;
- if (obj == null)
- return false;
- if (getClass() != obj.getClass())
- return false;
- Employee other = (Employee) obj;
- if (empId != other.empId)
- return false;
- if (empName == null) {
- if (other.empName != null)
- return false;
- } else if (!empName.equals(other.empName))
- return false;
- return true;
- }
- }
What is a data class in Kotlin? What is a data class in Kotlin?
We frequently create classes whose main purpose is to hold data. In Kotlin, this is called a data class and is marked as data
:
data class User(val name: String, val age: Int)
To ensure consistency and meaningful behavior of the generated code, data classes have to fulfill the following requirements:
- The primary constructor needs to have at least one parameter;
- All primary constructor parameters need to be marked as val or var;
- Data classes cannot be abstract, open, sealed or inner;
How would you override default getter for Kotlin data class? How would you override default getter for Kotlin data class?
Given the following Kotlin class:
data class Test(val value: Int)
How would I override the Int getter so that it returns 0
if the value negative?
Have your business logic that creates the data class alter the value to be 0 or greater before calling the constructor with the bad value. This is probably the best approach for most cases.
Don't use a
data class
. Use a regularclass
.class Test(value: Int) { val value: Int = value get() = if (field < 0) 0 else field override fun equals(other: Any?): Boolean { if (this === other) return true if (other !is Test) return false return true } override fun hashCode(): Int { return javaClass.hashCode() } }
Create an additional safe property on the object that does what you want instead of having a private value that's effectively overriden.
data class Test(val value: Int) { val safeValue: Int get() = if (value < 0) 0 else value }
What is a data class in Kotlin?
Data classes are those classes which are made just to store some data. In Kotlin, it is marked as data. The following is an example of the same:
data class Developer(val name: String, val age: Int)
When we mark a class as a data class, you don’t have to implement or create the following functions like we do in Java: hashCode()
, equals()
, toString()
, copy()
. The compiler automatically creates these internally, so it also leads to clean code. Although, there are few other requirements that data classes need to fulfill.
Learn more about data class from MindOrks blog.
Aaaa
Sealed Class
What are Kotlin Sealed Classes?
Sealed classes are another extremely useful feature of this modern-day programming language. They can be used to restrict the inheritance hierarchy of a class. All you need to do is define a class as sealed, and nobody will be able to create subclasses that belong to this sealed class.
These classes will come in handy when you use them inside when expressions. If you can perfectly cover all possible cases, you won’t have to use the else clause. However, remember that sealed classes are abstract by themselves and you can not instantiate one directly.
How to declare a sealed class?
Just put the sealed modifier before the name of the class.
Example
- sealed class Car {
- data class Maruti(val speed: Int) : Car()
- data class Bugatti(val speed: Int, val boost: Int) : Car()
- object NotACar : Car()
- }
The benefit of using sealed classes comes into play when you use them in an expression.
What are the benefits of using a Sealed Class over Enum?
Sealed classes give us the flexibility of having different types of subclasses and also containing the state. The important point to be noted here is the subclasses that are extending the Sealed classes should be either nested classes of the Sealed class or should be declared in the same file as that of the Sealed class.
Learn more about Sealed classes from MindOrks blog.
Kotlin Object
How to create Singleton classes?
To use the singleton pattern for our class we must use the keyword object
object MySingletonClass
An object
cannot have a constructor set. We can use the init block inside it though.
Rewrite this code in Kotlin Rewrite this code in Kotlin
Can you rewrite this Java code in Kotlin?
public class Singleton {
private static Singleton instance = null;
private Singleton(){
}
private synchronized static void createInstance() {
if (instance == null) {
instance = new Singleton();
}
}
public static Singleton getInstance() {
if (instance == null) createInstance();
return instance;
}
Using Kotlin:
object Singleton
How to create a Singleton class in Kotlin?
A singleton class is a class that is defined in such a way that only one instance of the class can be created and is used where we need only one instance of the class like in logging, database connections, etc.
To create a Singleton class in Kotlin, you need to use the object keyword.
object AnySingletonClassName
Note: You can't use constructor in object, but you can use init.
Learn more about Singleton class from MindOrks blog.
How would you create a singleton with parameter in Kotlin? ☆☆☆
Answer: Because a Kotlin object
can’t have any constructor, you can’t pass any argument to it.
So look at this code from Google's architecture components sample code, which uses the also
function:
class UsersDatabase : RoomDatabase() {
companion object {
@Volatile private var INSTANCE: UsersDatabase? = null
fun getInstance(context: Context): UsersDatabase =
INSTANCE ?: synchronized(this) {
INSTANCE ?: buildDatabase(context).also { INSTANCE = it }
}
private fun buildDatabase(context: Context) =
Room.databaseBuilder(context.applicationContext,
UsersDatabase::class.java, "Sample.db")
.build()
}
}
Source: stackoverflow.com
Kotlin Companion Object
Does Kotlin have the static keyword? How to create static methods in Kotlin?
NO. Kotlin doesn’t have the static keyword.
To create static method in our class we use the companion object
.
Following is the Java code:
class A {
public static int returnMe() { return 5; }
}
The equivalent Kotlin code would look like this:
class A {
companion object {
fun a() : Int = 5
}
}
To invoke this we simply do: A.a()
.
What is the equivalent of Java static methods in Kotlin? What is the equivalent of Java static methods in Kotlin?
Place the function in the companion object.
class Foo {
public static int a() { return 1; }
}
will become:
class Foo {
companion object {
fun a() : Int = 1
}
}
// to run
Foo.a();
Another way is to solve most of the needs for static functions with package-level functions. They are simply declared outside a class in a source code file. The package of a file can be specified at the beginning of a file with the package keyword. Under the hood these "top-level" or "package" functions are actually compiled into their own class. In the above example, the compiler would create a class FooPackage with all of the top-level properties and functions, and route all of your references to them appropriately.
Consider:
package foo
fun bar() = {}
usage:
import foo.bar
What are companion objects in Kotlin?
In Kotlin, if you want to write a function or any member of the class that can be called without having the instance of the class then you can write the same as a member of a companion object inside the class.
To create a companion object, you need to add the companion
keyword in front of the object declaration.
The following is an example of a companion object in Kotlin:
class ToBeCalled {
companion object Test {
fun callMe() = println("You are calling me :)")
}
}
fun main(args: Array<String>) {
ToBeCalled.callMe()
}
What is the equivalent of Java static methods in Kotlin?
To achieve the functionality similar to Java static methods in Kotlin, we can use:
- companion object
- package-level function
- object
Read more about this from MindOrks blog.
What is a purpose of Companion Objects in Kotlin? What is a purpose of Companion Objects in Kotlin?
Unlike Java or C#, Kotlin doesn’t have static
members or member functions. If you need to write a function that can be called without having a class instance but needs access to the internals of a class, you can write it as a member of a companion object declaration inside that class.
class EventManager {
companion object FirebaseManager {
}
}
val firebaseManager = EventManager.FirebaseManager
The companion object is a singleton. The companion object is a proper object on its own, and can have its own supertypes - and you can assign it to a variable and pass it around. If you're integrating with Java code and need a true static member, you can annotate a member inside a companion object with @JvmStatic
.
How do You Create Static Methods in Kotlin?
Static methods are useful for a number of reasons. They allow programmers to prevent the copying of methods and allow working with them without creating an object first. Kotlin doesn’t feature the widely used static keyword found in Java. Rather, you’ll need to create a companion object. Below, we’re comparing the creation of static methods in both Java and Kotlin. Hopefully, they will help you understand them better.
class A { public static int returnMe() { return 5; } // Java } class A { companion object { fun a() : Int = 5 // Kotlin } }
Why is there no static keyword in Kotlin?
The main advantage of this is that everything is an object. Companion objects can inherit from other classes or implement interfaces and generally behave like any other singleton.
In Java, static members are treated very differently than object members. This means that you can’t do things like implementing an interface or putting your class “instance” into a map or pass it as a parameter to a method that takes Object. Companion objects allow for these things. That’s the advantage.
Why is there no static keyword in Kotlin? ☆☆☆☆
Answer: The main advantage of this is that everything is an object. Companion objects can inherit from other classes or implement interfaces and generally behave like any other singleton.
In Java, static members are treated very differently than object members. This means that you can't do things like implementing an interface or putting your class "instance" into a map or pass it as a parameter to a method that takes Object. Companion objects allow for these things. That's the advantage.
Aaaaa
Extension Function
How do you think extension functions are useful ?
Extension functions helps to extend a class with new functionality without having to inherit from the class. Also you may use them like an inbuilt function for the class throughout the application.
Reference – Kotlin Extension Functions
Does Kotlin provide any additional functionalities for standard Java packages or standard Java classes?
Ofcourse, Yes. Kotlin uses the concept of extension functions, that we already talked about, to build some useful and more widely used functions among developers directly into the Kotlin library.
Explain the use of extension functions
Extension functions are beneficial for extending class without the need to inherit from the class.
Give me name of the extension methods Kotlin provides to java.io.File
- bufferedReader(): Use for reading contents of a file into BufferedReader
- readBytes() : Use for reading contents of file to ByteArray
- readText(): Use of reading contents of file to a single String
- forEachLine() : Use for reading a file line by line in Kotlin
- readLines(): Use to reading lines in file to List
List out some of the extension methods in kotlin?
Ans: Some of the extension methods are:
- read Text(): Helps to read content in the files to a single string.
- buffer Reader(): It is used to read contents of the file to buffer reader
- read each line(): It reads each line by line in the file
- readlines(): It helps to read lines of file for listing
What are extension functions in Kotlin?
Extension functions are like extensive properties attached to any class in Kotlin. By using extension functions, you can add some methods or functionalities to an existing class even without inheriting the class. For example: Let's say, we have views where we need to play with the visibility of the views. So, we can create an extension function for views like,
fun View.show() {
this.visibility = View.VISIBLE
}
fun View.hide() {
this.visibility = View.GONE
}
and to use it we use, like,
toolbar.hide()
How are extensions resolved in Kotlin and what doest it mean? ☆☆☆
Answer: Extensions do not actually modify classes they extend. By defining an extension, you do not insert new members into a class, but merely make new functions callable with the dot-notation on variables of this type.
The extension functions dispatched statically. That means the extension function which will be called is determined by the type of the expression on which the function is invoked, not by the type of the result of evaluating that expression at runtime. In short, they are not virtual by receiver type.
Consider:
open class BaseClass
class DerivedClass : BaseClass()
fun BaseClass.someMethod(){
print("BaseClass.someMethod")
}
fun DerivedClass.someMethod(){
print("DerivedClass.someMethod")
}
fun printMessage(base : BaseClass){
base.someMethod()
}
printMessage(DerivedClass())
This will print
BaseClass.someMethod
because the extension function being called depends only on the declared type of the parameter base
in printMessage
method, which is the BaseClass
class. This is different from runtime polymorphism as here it is resolved statically but not at the runtime.
What do you understand about function extension in the context of Kotlin? Explain.
In Kotlin, we can add or delete method functionality using extensions, even without inheriting or altering them. Extensions are statistically resolved. It provides a callable function that may be invoked with a dot operation, rather than altering the existing class.
// KOTLIN
class Sample {
var str : String = "null"
fun printStr() {
print(str)
}
}
fun main(args: Array<String>) {
var a = Sample()
a.str = "Interview"
var b = Sample()
b.str = "Bit"
var c = Sample()
c.str = a.add(b)
c.printStr()
}
// function extension
fun Sample.add(a : Sample):String{
var temp = Sample()
temp.str = this.str + " " +a.str
return temp.str
}
Output:-
Interview Bit
Explanation:-
We don't have a method named "addStr" inside the "Sample" class in the preceding example, but we are implementing the same method outside of the class. This is all because of function extension.
What do you understand about function extension in the context of Kotlin? Explain.
In Kotlin, we can add or delete method functionality using extensions, even without inheriting or altering them. Extensions are statistically resolved. It provides a callable function that may be invoked with a dot operation, rather than altering the existing class.
Function Extension - Kotlin allows users to specify a method outside of the main class via function extension. We'll see how the extension is implemented at the functional level in the following example:
// KOTLIN
class Sample {
var str : String = "null"
fun printStr() {
print(str)
}
}
fun main(args: Array<String>) {
var a = Sample()
a.str = "Interview"
var b = Sample()
b.str = "Bit"
var c = Sample()
c.str = a.add(b)
c.printStr()
}
// function extension
fun Sample.add(a : Sample):String{
var temp = Sample()
temp.str = this.str + " " +a.str
return temp.str
}
Output:-
Interview Bit
Explanation:-
We don't have a method named "addStr" inside the "Sample" class in the preceding example, but we are implementing the same method outside of the class. This is all because of function extension.
In Kotlin, we can add or delete method functionality using extensions, even without inheriting or altering them. Extensions are statistically resolved. It provides a callable function that may be invoked with a dot operation, rather than altering the existing class.
Function Extension - Kotlin allows users to specify a method outside of the main class via function extension. We'll see how the extension is implemented at the functional level in the following example:
// KOTLIN
class Sample {
var str : String = "null"
fun printStr() {
print(str)
}
}
fun main(args: Array<String>) {
var a = Sample()
a.str = "Interview"
var b = Sample()
b.str = "Bit"
var c = Sample()
c.str = a.add(b)
c.printStr()
}
// function extension
fun Sample.add(a : Sample):String{
var temp = Sample()
temp.str = this.str + " " +a.str
return temp.str
}
Output:-
Interview Bit
Explanation:-
We don't have a method named "addStr" inside the "Sample" class in the preceding example, but we are implementing the same method outside of the class. This is all because of function extension.
Kotlin Generics
What are Reified types in Kotlin?
When you are using the concept of Generics to pass some class as a parameter to some function and you need to access the type of that class, then you need to use the reified keyword in Kotlin.
For example:
inline fun <reified T> genericsExample(value: T) {
println(value)
println("Type of T: ${T::class.java}")
}
fun main() {
genericsExample<String>("Learning Generics!")
genericsExample<Int>(100)
}
Learn more about Reified keyword from MindOrks blog.
How does the reified keyword in Kotlin work
In an ordinary generic function like myGenericFun
, you can't access the type T
because it is, like in Java, erased at runtime and thus only available at compile time. Therefore, if you want to use the generic type as a normal Class
in the function body you need to explicitly pass the class as a parameter like the parameter c
in the example.
fun <T> myGenericFun(c: Class<T>)
By marking a type as reified
, we’ll have the ability to use that type within the function.
As for a real example, in Java, when we call startActivity
, we need to specify the destination class as a parameter. The Kotlin way is:
inline fun <reified T : Activity> Activity.startActivity() {
startActivity(Intent(this, T::class.java))
}
You can only use reified
in combination with an inline function. Such a function makes the compiler copy the function's bytecode to every place where the function is being used (the function is being "inlined"). When you call an inline function with reified type, the compiler knows the actual type used as a type argument and modifies the generated bytecode to use the corresponding class directly. Therefore calls like myVar is T
become myVar is String
(if the type argument were String
) in the bytecode and at runtime.
aaaaa
Comments
Post a Comment